How To Make Animation In Java Bluej
Animation
last modified July twenty, 2020
In this office of the Java 2d games tutorial, we volition work with animation.
Animation
Blitheness is a rapid display of sequence of images which creates an illusion of motion. Nosotros will animate a star on our Board. Nosotros will implement the movement in 3 basic means. We volition use a Swing timer, a standard utility timer, and a thread.
Animation is a circuitous subject in game programming. Java games are expected to run on multiple operating systems with unlike hardware specifications. Threads requite the about accurate timing solutions. However, for our elementary 2D games, other two options can exist an option too.
Swing timer
In the first example we will use a Swing timer to create animation. This is the easiest but likewise the least effective style of animating objects in Java games.
SwingTimerEx.coffee
packet com.zetcode; import coffee.awt.EventQueue; import javax.swing.JFrame; public class SwingTimerEx extends JFrame { public SwingTimerEx() { initUI(); } private void initUI() { add(new Board()); setResizable(fake); pack(); setTitle("Star"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { EventQueue.invokeLater(() -> { SwingTimerEx ex = new SwingTimerEx(); ex.setVisible(true); }); } }
This is the main class for the code example.
setResizable(false); pack();
The setResizable()
sets whether the frame tin be resized. The pack()
method causes this window to be sized to fit the preferred size and layouts of its children. Note that the gild in which these two methods are called is of import. (The setResizable()
changes the insets of the frame on some platforms; calling this method later the pack()
method might atomic number 82 to incorrect results—the star would not go precisely into the right-bottom border of the window.)
Board.java
packet com.zetcode; import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.Paradigm; import java.awt.Toolkit; import coffee.awt.issue.ActionEvent; import coffee.awt.result.ActionListener; import javax.swing.ImageIcon; import javax.swing.JPanel; import javax.swing.Timer; public class Board extends JPanel implements ActionListener { individual last int B_WIDTH = 350; individual final int B_HEIGHT = 350; private final int INITIAL_X = -twoscore; private final int INITIAL_Y = -forty; individual final int Filibuster = 25; individual Image star; private Timer timer; private int x, y; public Board() { initBoard(); } private void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = 2.getImage(); } private void initBoard() { setBackground(Color.BLACK); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); x = INITIAL_X; y = INITIAL_Y; timer = new Timer(DELAY, this); timer.start(); } @Override public void paintComponent(Graphics g) { super.paintComponent(chiliad); drawStar(g); } individual void drawStar(Graphics g) { g.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); } @Override public void actionPerformed(ActionEvent e) { x += 1; y += one; if (y > B_HEIGHT) { y = INITIAL_Y; 10 = INITIAL_X; } repaint(); } }
In the Board
class we move a star that from the upper-left corner to the correct-bottom corner.
private final int B_WIDTH = 350; individual final int B_HEIGHT = 350; private concluding int INITIAL_X = -twoscore; individual final int INITIAL_Y = -twoscore; private terminal int Delay = 25;
Five constants are defined. The first two constants are the lath width and height. The third and fourth are the initial coordinates of the star. The last one determines the speed of the animation.
private void loadImage() { ImageIcon 2 = new ImageIcon("src/resource/star.png"); star = ii.getImage(); }
In the loadImage()
method we create an instance of the ImageIcon
class. The image is located in the project directory. The getImage()
method will render the the Epitome
object from this form. This object will be drawn on the lath.
timer = new Timer(DELAY, this); timer.get-go();
Here we create a Swing Timer
course and call its beginning()
method. Every Filibuster
ms the timer will call the actionPerformed()
method. In order to use the actionPerformed()
method, nosotros must implement the ActionListener
interface.
@Override public void paintComponent(Graphics k) { super.paintComponent(thousand); drawStar(thousand); }
Custom painting is done in the paintComponent()
method. Annotation that we also call the paintComponent()
method of its parent. The bodily painting is delegated to the drawStar() method.
private void drawStar(Graphics g) { g.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); }
In the drawStar() method, we draw the paradigm on the window with the usage of the drawImage()
method. The Toolkit.getDefaultToolkit().sync()
synchronises the painting on systems that buffer graphics events. Without this line, the blitheness might non exist smooth on Linux.
@Override public void actionPerformed(ActionEvent due east) { x += 1; y += 1; if (y > B_HEIGHT) { y = INITIAL_Y; 10 = INITIAL_X; } repaint(); }
The actionPerformed()
method is repeatedly chosen by the timer. Inside the method, we increase the x and y values of the star object. Then we call the repaint()
method which will cause the paintComponent()
to be called. This way we regularly repaint the Board
thus making the animation.
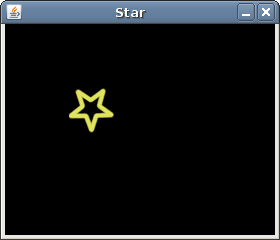
Utility timer
This is very similar to the previous mode. Nosotros use the java.util.Timer
instead of the javax.Swing.Timer
. For Coffee Swing games this manner is more authentic.
UtilityTimerEx.java
package com.zetcode; import java.awt.EventQueue; import javax.swing.JFrame; public class UtilityTimerEx extends JFrame { public UtilityTimerEx() { initUI(); } private void initUI() { add(new Board()); setResizable(fake); pack(); setTitle("Star"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void master(String[] args) { EventQueue.invokeLater(() -> { JFrame ex = new UtilityTimerEx(); ex.setVisible(true); }); } }
This is the principal grade.
Board.java
package com.zetcode; import coffee.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.Image; import coffee.awt.Toolkit; import coffee.util.Timer; import java.util.TimerTask; import javax.swing.ImageIcon; import javax.swing.JPanel; public class Board extends JPanel { individual concluding int B_WIDTH = 350; private last int B_HEIGHT = 350; private concluding int INITIAL_X = -40; private final int INITIAL_Y = -forty; private final int INITIAL_DELAY = 100; individual concluding int PERIOD_INTERVAL = 25; private Image star; private Timer timer; private int x, y; public Board() { initBoard(); } individual void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = ii.getImage(); } individual void initBoard() { setBackground(Color.Blackness); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); x = INITIAL_X; y = INITIAL_Y; timer = new Timer(); timer.scheduleAtFixedRate(new ScheduleTask(), INITIAL_DELAY, PERIOD_INTERVAL); } @Override public void paintComponent(Graphics g) { super.paintComponent(one thousand); drawStar(g); } private void drawStar(Graphics 1000) { one thousand.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); } private class ScheduleTask extends TimerTask { @Override public void run() { x += 1; y += ane; if (y > B_HEIGHT) { y = INITIAL_Y; x = INITIAL_X; } repaint(); } } }
In this example, the timer will regularly call the run()
method of the ScheduleTask
class.
timer = new Timer(); timer.scheduleAtFixedRate(new ScheduleTask(), INITIAL_DELAY, PERIOD_INTERVAL);
Here we create a timer and schedule a job with a specific interval. There is an initial delay.
@Override public void run() { ... }
Each 10 ms the timer will call this run()
method.
Thread
Animating objects using a thread is the most effective and accurate manner of blitheness.
ThreadAnimationEx.coffee
package com.zetcode; import java.awt.EventQueue; import javax.swing.JFrame; public grade ThreadAnimationEx extends JFrame { public ThreadAnimationEx() { initUI(); } private void initUI() { add together(new Board()); setResizable(faux); pack(); setTitle("Star"); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } public static void main(String[] args) { EventQueue.invokeLater(() -> { JFrame ex = new ThreadAnimationEx(); ex.setVisible(truthful); }); } }
This is the main class.
Board.coffee
package com.zetcode; import java.awt.Colour; import coffee.awt.Dimension; import java.awt.Graphics; import java.awt.Epitome; import coffee.awt.Toolkit; import javax.swing.ImageIcon; import javax.swing.JOptionPane; import javax.swing.JPanel; public class Board extends JPanel implements Runnable { individual concluding int B_WIDTH = 350; private final int B_HEIGHT = 350; private final int INITIAL_X = -40; private final int INITIAL_Y = -40; individual final int DELAY = 25; private Epitome star; private Thread animator; private int x, y; public Board() { initBoard(); } individual void loadImage() { ImageIcon ii = new ImageIcon("src/resources/star.png"); star = two.getImage(); } private void initBoard() { setBackground(Color.BLACK); setPreferredSize(new Dimension(B_WIDTH, B_HEIGHT)); loadImage(); 10 = INITIAL_X; y = INITIAL_Y; } @Override public void addNotify() { super.addNotify(); animator = new Thread(this); animator.start(); } @Override public void paintComponent(Graphics g) { super.paintComponent(1000); drawStar(k); } individual void drawStar(Graphics chiliad) { one thousand.drawImage(star, x, y, this); Toolkit.getDefaultToolkit().sync(); } private void cycle() { ten += ane; y += one; if (y > B_HEIGHT) { y = INITIAL_Y; x = INITIAL_X; } } @Override public void run() { long beforeTime, timeDiff, slumber; beforeTime = System.currentTimeMillis(); while (true) { bicycle(); repaint(); timeDiff = Arrangement.currentTimeMillis() - beforeTime; sleep = DELAY - timeDiff; if (sleep < 0) { sleep = ii; } attempt { Thread.sleep(slumber); } catch (InterruptedException e) { String msg = String.format("Thread interrupted: %s", east.getMessage()); JOptionPane.showMessageDialog(this, msg, "Error", JOptionPane.ERROR_MESSAGE); } beforeTime = Organization.currentTimeMillis(); } } }
In the previous examples, we executed a task at specific intervals. In this instance, the animation volition take place inside a thread. The run()
method is chosen just once. This is why why we have a while loop in the method. From this method, we call the bicycle()
and the repaint()
methods.
@Override public void addNotify() { super.addNotify(); animator = new Thread(this); animator.outset(); }
The addNotify()
method is chosen afterward our JPanel
has been added to the JFrame
component. This method is frequently used for various initialisation tasks.
We want our game run smoothly, at abiding speed. Therefore we compute the arrangement time.
timeDiff = Organisation.currentTimeMillis() - beforeTime; sleep = Filibuster - timeDiff;
The wheel()
and the repaint()
methods might have different time at diverse while cycles. We calculate the time both methods run and subtract information technology from the Delay
constant. This way we desire to ensure that each while cycle runs at constant fourth dimension. In our instance, it is DELAY
ms each cycle.
This office of the Java 2D games tutorial covered animation.
Source: https://zetcode.com/javagames/animation/
Posted by: kennerhishmad.blogspot.com
0 Response to "How To Make Animation In Java Bluej"
Post a Comment